- What Will You Learn?
- Getting Ready for WordPress Development
- PHP for WordPress
- Introduction
- What is PHP?
- PHP Review:
- Writing Some Basic PHP
- PHP Programming Basics
- PRACTICE – PHP Basics
- WordPress PHP Coding Standards
- Different Types of PHP Files in WordPress
- The Loop
- PRACTICE – The Loop
- Template Tags
- PRACTICE – Template Tags
- WordPress Conditionals
- PRACTICE – Conditional Tags
- WordPress Hooks
- PRACTICE – WordPress Hooks
- PHP for WordPress Review
- Child Themes and Starter Themes
WordPress Theme & Plugin Development
home / blog / WordPress Theme & Plugin Development
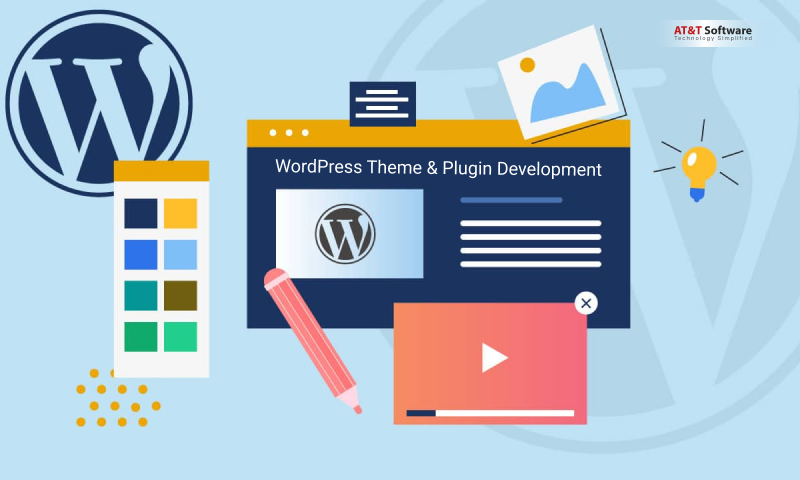
What Will You Learn?
- What Will You Learn?
- Getting Ready for WordPress Development
- PHP for WordPress
- Introduction
- What is PHP?
- PHP Review:
- Writing Some Basic PHP
- PHP Programming Basics
- PRACTICE – PHP Basics
- WordPress PHP Coding Standards
- Different Types of PHP Files in WordPress
- The Loop
- PRACTICE – The Loop
- Template Tags
- PRACTICE – Template Tags
- WordPress Conditionals
- PRACTICE – Conditional Tags
- WordPress Hooks
- PRACTICE – WordPress Hooks
- PHP for WordPress Review
- Child Themes and Starter Themes
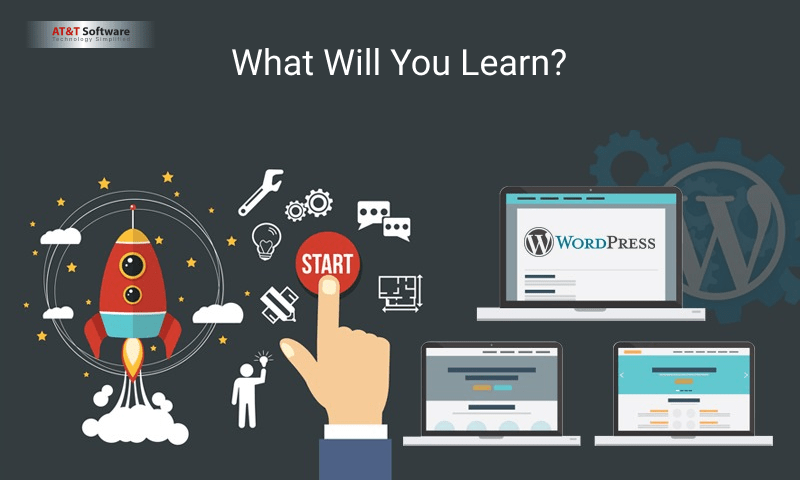
- PHP for WordPress – The Loop, Conditionals, Hooks, and More!
- How to Work with Starter Themes Like Pro
- The Ins and Outs of the Template Hierarchy – Always Know What File to Customize!
- How To Enqueue and Work with JavaScript and CSS in Themes
- The Complete List of Template Tags That is Needed to Use When Customizing and Extending Themes
- A Deep Understanding of How to Use Action and Filter Hooks to Programmatically Control WordPress
- A Solid Starter Template For Building Your Own WordPress Plugins
- Standard Practices and Techniques for Building Custom WordPress Plugins
Points | Description |
---|---|
Getting Ready for WordPress Development | 9 lectures, 49min |
PHP for WordPress | 16 lectures, 1hr 32min |
Child Themes and Starter Themes | 8 lectures, 37min |
The Template Hierarchy | 42 lectures, 4hr 55min |
Template Tags | 45 lectures, 3hr 59min |
Action and Filter Hooks in WordPress | 34 lectures, 2hr 23min |
Plugin Development | 22 lectures, 1hr 54min |
Getting Ready for WordPress Development
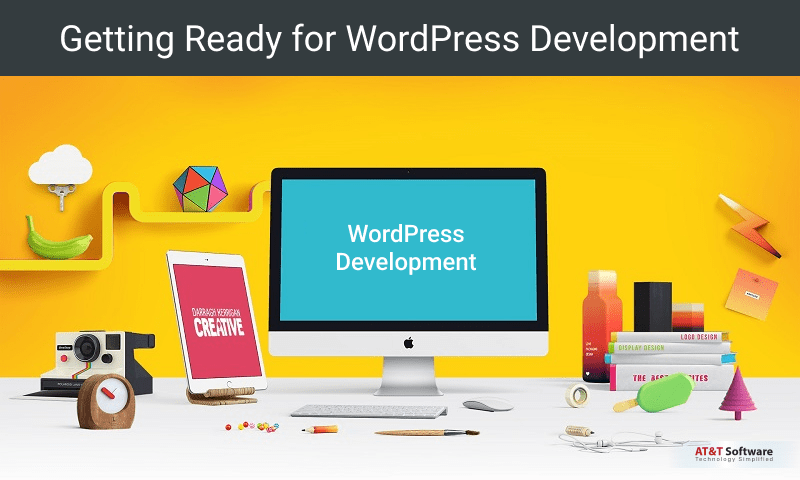
Course Introduction
Here we introduce the course structure and an overall learning curriculum. This course needs your proficiency with HTML and CSS, so we recommend you to learn that first.
Topics Covered By The Course:
- How to set up your local environment
- How to setup your hosting environment
- PHP for WordPress- The Loop, Conditionals, Tags, Hooks, etc
- How to work with Child Themes and Starter Themes
- The Template Hierarchy and Template Tags
- Action and Filter Hooks
- How to Start Building Plugins
- Internal WordPress REST APIs
The mentor will give you a real-time demonstration to build a theme and plugin and the candidates need to follow this intensively. All the knowledge and details about building one’s own themes and plugins will be explained by the faculty.
Next up, we’ll look at how to set up our local WordPress environment to run WordPress on the computer.
Setting Up WordPress Locally
Local WordPress Development refers to running WordPress on your computer and editing the files locally, on your computer, rather than using a hosted version of WordPress to develop with.
We look at a few different options for locally running WordPress on the computers:
1. DesktopServer from ServerPress
In this lesson, we understand how to run WordPress locally using DesktopServer from ServerPress.
2. Local from Flywheel
Here we learn the ways to run WordPress locally using Local from Flywheel.
Editing WordPress Files Locally
Finding the WordPress files that you will need to edit when you work with Desktop and Local, is all explained in the editing process.
Introduction to Staging
Pulling from Production to Staging to Local
Pushing from Local to Staging to Production
PHP for WordPress
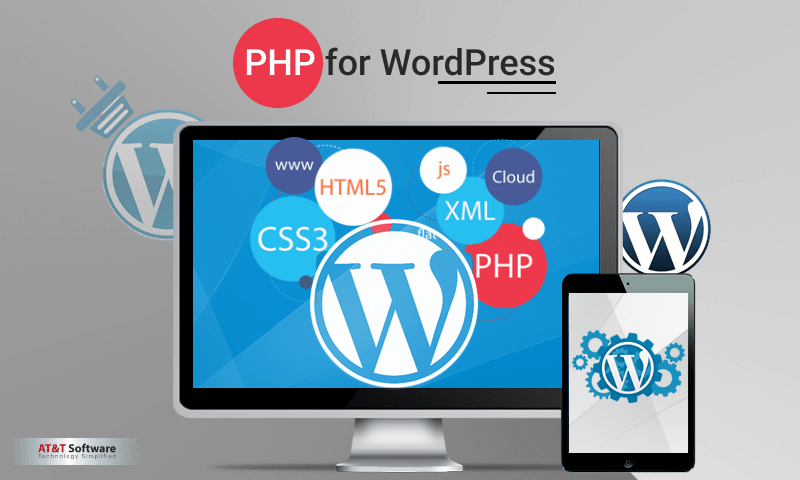
Introduction
Here we introduce the section on ‘PHP for WordPress’ and go over on what we will learn in forth-coming studies:
A quick glimpse on what we will learn:
- Explain what PHP is and how it works.
- Write some PHP and learn the basics of the language
- Introduce important WordPress specific PHP things that are important, for example-The Loop, Template Tags, and Hooks
What is PHP?
PHP stands for “PHP: Hypertext Preprocessor” and runs before the HTML for a web page is generated. PHP generally works in WordPress and can be used outside of WordPress as well. It is used to build sites and applications.
PHP Review:
- PHP is a programming language.
- It runs on the server before HTML is sent to the browser
- PHP is used extensively in WordPress itself and WordPress Development
- PHP can also be used outside of WordPress in other applications and websites
Writing Some Basic PHP
The basic writing of PHP is introduced here.
Aspects Covered:
- Opening an index.php file
- Writing a variable
- Echoing a variable
- Echoing a string of text
- Concatenating or combining variables and strings.
PHP Programming Basics
In the text lessons, we outline some of the basics related to working with PHP that we must know going forward. Read through and absorb what you can, and in the next lesson, we will look at putting some of this into practice.
PRACTICE – PHP Basics
Here we work on the following practice exercise:
- Activate theme 1.4 PHP Basics (Starter)
- Create an array of post titles
- Loop through the variety of posts
- Inside the Loop, call a display_title() function and pass the title as a parameter.
- Have the display_title() function echo the title in an <h3> tag
Try practicing this on your own before checking out the completed solution and the walk-through in the video.
WordPress PHP Coding Standards
In this lesson, we learn about the WordPress Coding Standards. These are guidelines and suggestions for how to write and format your PHP when working with WordPress.
You can access the WordPress PHP Coding Standards here:
https://make.wordpress.org/core/handbook/best-practices/coding-standards/php/
The WordPress Coding Standards contain information around the following topics:
- Single and Double Quotes
- Indentation
- Brace Style
- Use elseif, not else if
- Regular Expressions
- No Shorthand PHP Tags
- Remove Trailing Spaces
- Space Usage
- Formatting SQL statements
- Database Queries
- Naming Conventions
- Self-Explanatory Flag Values for Function Arguments
- Interpolation for Naming Dynamic Hooks
- Ternary Operator
- Yoda Conditions
- Clever Code
- Error Control Operator @
- Don’t extract ()
Not all of these may make sense to you at this point, so try reading through these now and checking back on them again at different points in the course. You will master these all in the due course.
Different Types of PHP Files in WordPress
In general, you will find three available types of PHP files in WordPress:
WordPress Core Files
These control how WordPress works, not edited, but exciting and possibly helpful to read through or study.
WordPress Theme Files
These control how themes work and display content. When you are building or customizing a child theme, you will edit these files.
WordPress Plugin Files
These are used when building plugins. If you are writing your own plugin or extending another plugin, you will edit these files, but you would not generally directly edit the code of another plugin.
Include Files
Small PHP files included in larger files appear in Core, Theme, and Plugin files.
You will likely come across and write them yourself.
The Loop
The Loop combines a conditional statement and Loop that intelligently gathers and prepares post or page content to display.
It consists of the following:
- A PHP If Statement
- A PHP While Loop
- A special WordPress function calls to get the correct post or page (or custom post etc.)
A basic loop looks like this:
<?php if ( have_posts() ) : while ( have_posts() ) : the_post(); ?>
<h2><?php the_title(); ?></h2>
<?php the_content(); ?>
<?php endwhile; else: ?>
<h2><?php esc_html_e( ‘404 Error’, ‘phpforwp’ ); ?></h2>
<p><?php esc_html_e( ‘Sorry, content not found.’, ‘phpforwp’ ); ?></p>
<?php endif; ?>
Notice that since the PHP code is inside various self-contained PHP blocks, it is also possible to use HTML inside the Loop.
It is also possible, though, to have a pure PHP loop without any HTML being included between PHP blocks:
<?php if ( have_posts() ) : while ( have_posts() ) : the_post();
the_title( ’<h1>’, ’</h1>’ );
the_content();
endwhile; else:
_e( ‘Sorry, no pages matched your criteria.’, ‘textdomain’ );
endif; ?>
It is also possible to customize the Loop, which we will learn at a later time in the course.
PRACTICE – The Loop
Now it’s time to practice using PHP Loop on your own:
- Activate theme 1.8 – The Loop (Starter)
- Open index.php
- Code out The Loop
- Use the_title and the_content to display the content
- Display a 404 message using _e() if no content is available
- Open a page or post from WP Admin to test
Try tackling this yourself, then follow along with me as I show you the approach I took.
Template Tags
Template tags are special functions that allow us to get information and content from WordPress easily.
Some popular template tags include:
- get_header()
- get_footer()
- get_sidebar()
- get_template_part()
- wp_login_form()
- bloginfo()
- the_title()
- get_the_title()
- the_content()
- the_author()
- the_category()
- the_tags()
- comment_author()
- the_post_thumbnail()
- the_permalink()
- edit_post_link()
- site_url()
- wp_nav_menu()
PRACTICE – Template Tags
Now that we’ve learned about template tags, it’s time for you to tackle some practice on your own:
- Activate theme 1.10 – Template Tags (Starter)
- Open index.php
- Add template tags inside The Loop
- Add template tags outside The Loop
- See how many different template tags you can get working!
After you take a stab on your own, you can follow along with my solution 🙂
WordPress Conditionals
Conditional Tags are WordPress functions that return true when certain conditions are met.
Some common conditional tags include:
- is_front_page()
- is_home()
- is_admin()
- is_single()
- is_single( ‘slug’ )
- is_single( [ ‘slug-1’, ‘slug-2’, ‘slug-3’ ] )
- is_singular()
- get_post_type()
- has_excerpt()
- is_page()
- is_page( ‘slug’ )
- is_page( [ ‘slug-1’, ‘slug-2’, ‘slug-3’ ] )
- is_page_template( ‘custom.php’ )
- comments_open()
- is_category()
- is_tag()
- is_archive()
- in_the_loop()
PRACTICE – Conditional Tags
Now that you’ve learned about Conditional Tags, let’s try practicing writing some:
- Activate theme 1.12 – Conditional Tags (Starter)
- Open index.php
- Try adding conditional tags.
- Open page from admin or visit site.dev/category site.dev/tag URL directly
- Might need && || !
WordPress Hooks
Hooks allow you to add custom code to existing software.
Two types of hooks exist in WordPress:
1. Action Hooks let you run your code when certain events take place in the WordPress run cycle.
2. Filter Hooks let you modify how content is displayed on a page or saved to the database.
Here is an example of an Action Hook in use:
“`
<?php
function my_theme_styles() {
wp_enqueue_style( ‘main-css’, get_stylesheet_uri() );
}
add_action( ‘wp_enqueue_scripts’, ‘my_theme_styles’ );
?>
“`
Here is an example of a Filter Hook in use:
“`
<?php
function my_read_more_link( $excerpt ) {
return $excerpt . ‘<a href=”‘ . get_permalink() . ‘”>Read more</a>’;
}
add_filter( ‘get_the_excerpt’, ‘my_read_more_link’, 10 );
?>
PRACTICE – WordPress Hooks
Now it’s time to practice writing some hooks on your own.
Try the following:
- Activate 1.14 – WordPress Hooks (Starter)
- Open the functions.php
- Try enqueueing the theme CSS via an Action Hook
- Try adding a Read More link to the_excerpt() with a filter.
PHP for WordPress Review
Some important points for review:
- PHP is a server-side programming language.
- Many Core, Plugin, and Theme files in WordPress are PHP.
- Some basic PHP is required for WordPress Development.
- WordPress provides The Loop, Template Tags, and Conditionals to make writing PHP easier.
- Action and Filter Hooks let us run our own code and modify data in WordPress programmatically.
Child Themes and Starter Themes
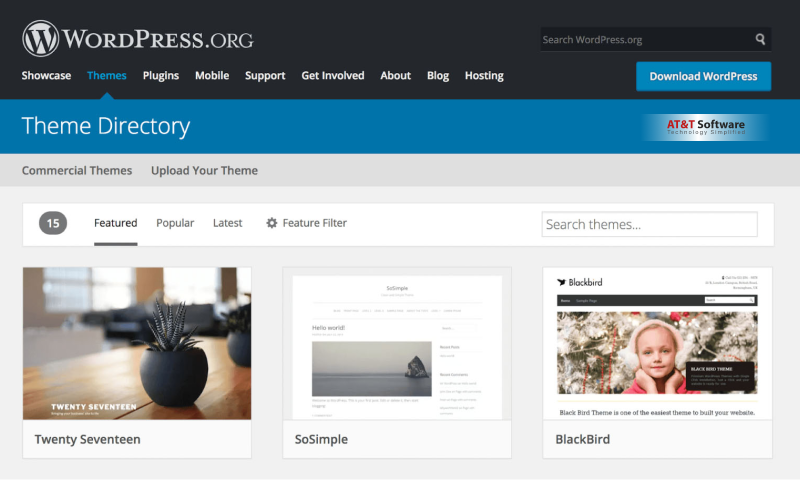
Child Themes vs Starter Themes
A Child Theme allows you to override another theme (parent theme) without making direct changes that are lost during updates.
A Starter Theme includes helpful files and functions for building themes from scratch. You usually edit starter themes directly, not using child themes.
Here is when to use each:
Child Theme – Customizing an existing theme
Starter Theme – Building sites from scratch.
Child Theme Basics
Child Theme
- Refers to parent theme in style.css file
- Include parent style in functions.php file
- Copies of any templates from Parent Theme you want to modify.
Some Other Aspects of Child Theme:
- Keeps all original files unedited
- Before loading a file, WordPress looks to see if it exists in Child Theme.
- Can be updated without affecting code in Child Theme
DEMO – Child Theme
In this lesson, we take a look at a child theme in action.
PRACTICE – Child Themes
Now it is time for you to practice making a child theme:
- Pick a WordPress theme
- Create a Child Theme for it
- Modify the CSS and one of the templates
- You don’t need to make it look better. Just get it working!
- Demo and Practice Child Theme files
Starter Theme Basics
Starter Theme Basics:
- Include standard theme template files.
- No CSS styles (but some include Bootstrap / Foundation)
- Extra functionality in functions.php
- Extra hooks in template files
- Follow modular file architecture
- Some include workflow tools
- Usually edited directly, not via child themes
DEMO – Underscore Starter Theme
PRACTICE – Starter Themes
In this lesson, I encourage you to go practice working with a starter theme on your own:
- Choose a starter theme (I recommend JointsWP)
- Install and Activate it
- Make a CSS change
- Make a change to one of the templates
WARNING: Not all starter themes are created equal
Child and Starter Themes Review
Child Theme Review:
- When customizing a theme, use a Child Theme
- When starting from scratch, use a Starter Theme
- It is also possible to start without child theme or starter theme!

I hope you enjoy reading this blog post.
Would you like to get expert advice? Schedule a Call
About Webrock Media
Webrock Media comes with an incredible team of website and mobile application developers who can customize the perfect solutions to transform your business. We think ourselves to be an ideal ‘Technology Simplified Destination’ as we know how to perfectly merge creativity and programming to build robust websites for our clients.
